It’s that time of the year again. Some of you just celebrated Thanksgiving, while the rest of the world only “celebrated” Black Friday. But I’m speaking about the 24 days before Christmas. Starting in 2015, I’ve set myself the goal to write one blog post per day. I managed to do that in 2015 and 2016 in German. In 2017, I did it in English as well in, but after only 10 day, I had to stop. Since then, I haven’t tried it again.
Restart of the advent calendar
This year, I will try to do it again. In the past editions, I hadn’t really planned all topic, if any. But this year, I was prepared a week early and already collected 24 potential topics to write about. I will focus on frontend topics and debugging. Every day, I will write about one of these four topics:
- Debugging
- JavaScript
- CSS
- HTML
The topics will be alternating, so you will get a debugging topic today, and then again in 4 days. The debugging topic came to mind, since we had some team learning session on Xdebug and looked at debugging in the browser as a follow-up. In my 6 blog posts on this topic, I will focus on the debugging in the browser.
Logging in the browser
The topic of debugging is very brought, and modern browsers offer numerous ways to debug things. So, let’s get started with something basic: logging things in JavaScript.
When you write some code in JavaScript, you might want to debug the code and see the value of certain things in the flow of a piece of code. In order to do this, you can use the console.log()
function:
console.log(some_variable);
You can add this line to a JavaScript file in your (external) JavaScript code you use in your project, but you can also use this in the browser console directly. All modern browsers do have such a JavaScript console. In Chrome, you can open them with “CTRL + SHIFT + J” (Windows and Linux).
Logging some values
After opening the “Console” tab in the DevTools, you can enter any JavaScript code in the “prompt” at the end of the console. Let’s debug something here:
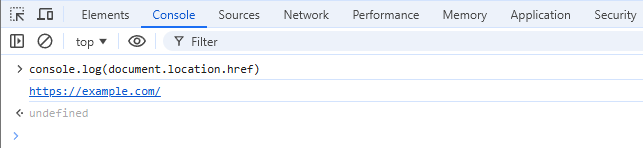
In this very simple example, we are debugging the value of the current URL of the site. The first line after our executed code is the output of the console.log()
function. The second line with the undefined
value is the response of the function itself.
If you are using the browser console, you don’t even need to use the console.log()
function. You could directly type the JavaScript code into the prompt and hit enter. The console.log()
function is usually used in some (external) script on your site:
const colors = ["red", "green", "blue"];
const capitalizedColors = colors.map(item => {
console.log(item);
return item.charAt(0).toUpperCase() + item.slice(1);
});
console.log(capitalizedColors);
Let’s take this sample code. We have an array with colors and want to capitalize the values. We use the map()
function on the array. Inside the function, we log the current item
and after we have run it, we log the resulting capitalizedColors
variable. The logging in the browser looks like this:
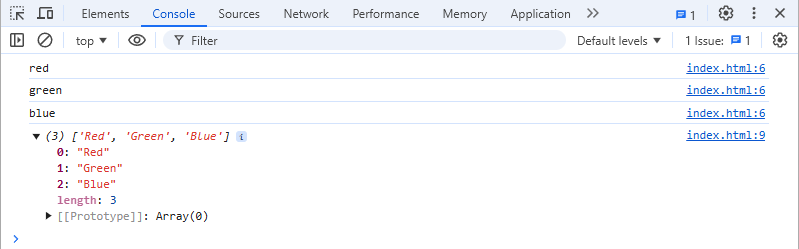
Since the array has three items, we see three lines with the value. At the end of each logged value, we see a reference index.html:6
, which indicated the filename and line in this file the logging was done. We also see the logged array, which I already expanded by clicking on the small arrow. Chrome will even show us the length of the array and the “[[Prototype]]” for the array. If we expand this, we can see all properties and functions of the array. Here we would also find the map()
function we just used.
Conclusion
That should be enough for today. Many of you will probably already know about this function. But did you know that there are more debugging functions available? We will take a closer look at some of them in the following blog posts in December.
I mainly use the Chrome DevTools, but you can also use the Firefox DevTools similarly. If you use Safari, Edge, Brave, or any other popular browser, just search for some resources on how to get started with those tools. So give it a try and debug some of your own JavaScript code.
[…] the very first advent calendar blog post this year, I’ve shown you how to “Getting started with JavaScript debugging“. Today, I want to show some more advanced techniques you can use to debug your […]