When we have to create more complex forms, we often use the Gravity Forms plugins. In one project, we wanted to use it to implement a form with multiple steps, each only having one radio group. Users should see the number of steps and the progress in the form. This is fairly simple, you can just add a “Page” and the other fields to these pages. When you insert the form into a post or page, you will get the following result:
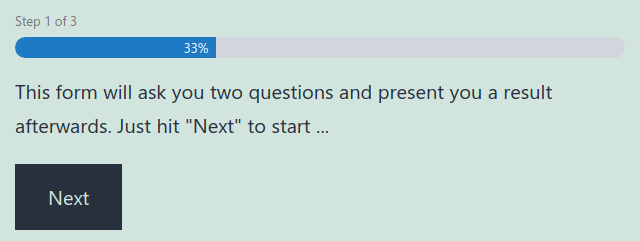
You can see the issue in this screenshot. The first pages does not have any form fields, but only a “HTML” field with an introduction text. But as it is a page of the form, the progress bar already starts counting.
Starting at page two
In order to fix this, we want to do two things. First we want to hide the progress bar from the first page. But then we would start with “Step 2 of 3” instead of “Step 1 of 2”, so we also have to update the text for the steps on all following pages.
Fortunately Gravity Forms offers a lot of hooks we can use. Hooks can either be used of all fields and form or just for specifics fields or forms, by adding the ID of the field or form to the end of the hook name. But as this is a bit unflexible, we first write a little helper function to check, if we are rendering the form we want to change the progress bar for:
function gf_pbc_is_customized_form( $form ) {
if ( 'processbar-customized' === $form['cssClass'] ) {
return true;
}
return false;
}
In this example I’ve added a CSS class processbar-customized
in the form settings. You could also use the form ID, title, or any other property.
Now we can change the start of the steps counter. This is fairly easy, as Gravity Forms offers a filter to start the steps at zero instead of one:
function gf_pbc_start_at_zero( $start_at_zero, $form ) {
return gf_pbc_is_customized_form( $form );
}
add_filter( 'gform_progressbar_start_at_zero', 'gf_pbc_start_at_zero', 10, 2 );
Inside of this function, we use our previous function to check, if we are rendering a form we want to start at zero.
Changing the progress bar text
Now we can hide the progress bar on the first page and change the text on all following pages. Here we use a different filter. The result may look like this:
function gf_pbc_progressbar_steps( $progress_bar, $form, $confirmation_message ) {
if ( ! gf_pbc_is_customized_form( $form ) ) {
return $progress_bar;
}
$current_page = GFFormDisplay::get_current_page( $form['id'] );
$max_page = GFFormDisplay::get_max_page_number( $form );
if ( 1 === $current_page ) {
$progress_bar = '';
} else {
$progress_bar = preg_replace_callback(
'#<p class="gf_progressbar_title">.*(gf_step_current_page?)(?s).*</p>#',
function ( $matches ) use ( $current_page, $max_page ) {
if ( ! isset( $matches[1] ) ) {
return $matches[0];
}
return '<p class="gf_progressbar_title">' . sprintf(
// translators: %1$d: number of page, %2$d: number of max_pages.
__( 'Question %1$d of %2$d', 'gf-pbc' ),
$current_page - 1,
$max_page - 1
) . '</p>';
},
$progress_bar
);
}
return $progress_bar;
}
add_filter( 'gform_progress_bar', 'gf_pbc_progressbar_steps', 10, 3 );
First we check again, if we want to change the rendering. If not, we just return the progress bar unchanged. Then we get the current page number and the max number of pages, we might need later. The condition then checks, if we are on the first page. In this case, we simply return an empty string, which will completely the progress HTML markup.
If we are on another page, we use a regex to alter the HTML of the page. Using regular expressions for HTML is not always ideal, but unfortunately we cannot change single parts of the progress bar HTML and copying the whole original file from Gravity Forms would be too much for this simple customization.
In the return string, we also the title with a prefix of “Question” instead of “Step”. Then we pass the current number and the max page number both one lower than the original value. With this customization, the progress bar will start with zero width and would have 100% width on the results page (where it is not showed by default).
Conclusion
Sometimes a project will have requirements that cannot be fulfilled with the settings of a plugin only. But better plugins will offer some hooks you can use to also solve such case. As usual, you can find the code of this bost post in a GIST and download it as a ZIP file from there to install it as a plugin.
Very helpful content. Is it possible to hide the progressbar only by custom CSS? like nth-child or something like this?
I’d say this is not possible. I can’t find any CSS class that tells the form which step is currently shown.