In the first debugging blog post, we’ve learned about the console.log()
method and how it works to debug scalar types or arrays. But there is a nicer way to log arrays or objects in the browser.
The console.table() function
As mentioned in the first blog post, the console
object has some more useful functions, and today we want to take a look at the table()
function.
Simple arrays
The function can be used to log arrays like this:
const colors = ["red", "green", "blue"];
console.table(colors);
This is the most basic example. We just use a one-dimensional (numerical) array. This is the result in the browser console:
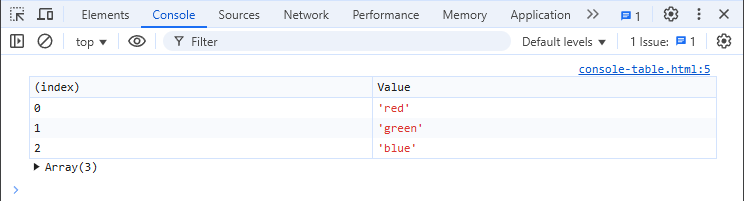
As you can see here, you get a table with two columns. The first one is the numerical “(index)” for the array, and the second one has the “Value” for each entry of the array. You will still always have the variable below the table, just as with console.log()
and you can “expand” it here, to investigate it further.
Multidimensional arrays
The function can not only be used with simple one dimensional array, but you can also use it for multidimensional ones:
const names = [
["Leia", "Organa"],
["Luke", "Skywalker"],
["Han", "Solo"],
];
console.table(names);
Now we have an array of (numerical) arrays and this is how it looks like in the console:
(index) | 0 | 1 |
---|---|---|
0 | 'Leia' | 'Organa' |
1 | 'Luke' | 'Skywalker' |
2 | 'Han' | 'Solo' |
We now have three columns. Since the “inner arrays” are numerical as well, we have column name “0” and “1” for them.
Logging objects
The function cannot only be used for arrays, but also for objects. The output depends on the structure of the objects.
Simple objects
Let’s create an object for one of the previous example:
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
const luke = new Person("Luke", "Skywalker");
console.table(luke);
And this is how that object would be logged into the browser console:
(index) | Value |
---|---|
firstName | 'Luke' |
lastName | 'Skywalker' |
Logging arrays of objects
How about logging an array with multiple objects of the same type? Again, an example like before:
const leia = new Person("Leia", "Organa");
const luke = new Person("Luke", "Skywalker");
const han = new Person("Han", "Solo");
console.table([leia, luke, han]);
This is the resulting table for this array of objects:
(index) | firstName | lastName |
---|---|---|
0 | 'Leia' | 'Organa' |
1 | 'Luke' | 'Skywalker' |
2 | 'Han' | 'Solo' |
As many of you might have expected, the column names are now the property names of the objects.
Logging multidimensional objects
The previous example was using a numerical array. But how about an object of objects? Here is an example:
const family = {};
family.mother = new Person("Padmé", "Amidala");
family.father = new Person("Anakin", "Skywalker");
family.daughter = new Person("Leia", "Organa");
family.son = new Person("Luke", "Skywalker");
console.table(family);
And the result of this will look like this:
(index) | firstName | lastName |
---|---|---|
mother | 'Padmé' | 'Amidala' |
father | 'Anakin' | 'Skywalker' |
daughter | 'Leia' | 'Organa' |
son | 'Luke' | 'Skywalker' |
That looks nice, right? Except for “(index)”, we have some good columns names and our index values also are not numerical anymore.
Logging only specific columns
The console.table()
function has a second parameter columns
, which is an array as well. It expects an array with the names of the columns/indices. So for our previous code, we could do this:
console.table(family, ["firstName"]);
And sure enough, this would be the logged results:
(index) | firstName |
---|---|
mother | 'Padmé' |
father | 'Anakin' |
daughter | 'Leia' |
son | 'Luke' |
Pretty neat, isn’t it? This can be really useful when debugging some larger objects with multiple properties.
Conclusion
Even though you can use the generic console.log()
function to debug and log arrays and objects, seeing them in a logged table, can make reading them a lot easier. You also don’t have to “expand” the tables.