You should usually use each of the basic web technologies for their main purposes: HTML for the markup, CSS for the styles and JavaScript for additional interactivity. But sometimes, those things cross into each other. There might be cases, in which you need to know the current rendered styles of an element. That’s what the topic today is about.
Get the computed styles of an element
The function name for that couldn’t be easier to remember: getComputedStyle()
. It’s a function of the window
object and gets passed the element we want the styles for:
const body = document.querySelector('body');
const bodyStyles = window.getComputedStyle(body);
const bodyFontSize = bodyStyles.getPropertyValue('font-size');
console.log(bodyFontSize); // 16px
To get a single CSS property, you use the getPropertyValue()
function on the CSSStyleDeclaration
object (in our case bodyStyles
), and tell it what property you want to get.
If you run this code in a completely empty HTML, you will probably get 16px
as the body font size, which is the default in most browsers, unless the size was changed in the operating system or browser.
But let’s see, where this can get more interesting. You don’t always have a fixed font width on an element, but might have one that is relative to other elements:
body {
font-size: 22px;
}
h1 {
font-size: 1.6em;
}
h1 small {
font-size: 75%;
}
Now we have a website with a <h1>
headline and some <small>
element inside of it. What would our resulting font size be? Let’s grab our calculators:
<body>
font-size: 22px<h1>
font-size: 22px * 1.6 = 35.2px<small>
inside<h1>
font-size: 35.2px * 0.75 = 26.4px
So we should get a font-size of 26,4px for the small text. Let’s see, if that is the case, by also checking it with the dev tools:
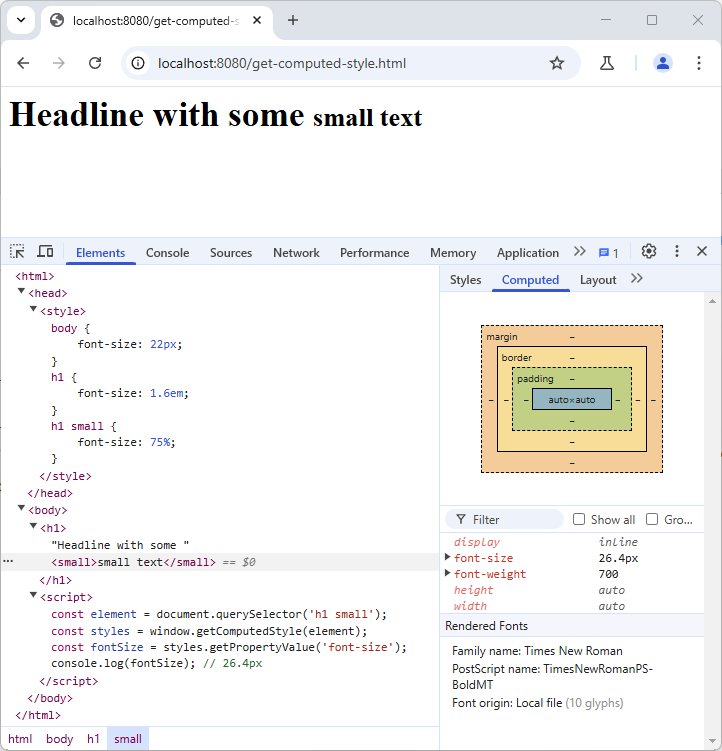
Here you can see a full example, with the modified selector. We have selected the <small>
element, and Chrome shows us the 26.4px font-size in the “Computed” section. As I wrote after the console.log()
call, this is also what we would get from JavaScript. Quite nice, isn’t it?
Getting styles from a pseudo-element
If an element has a pseudo-element, either specifically set or available by default, you can query styles for them. Let’s set some styles for the new ::marker
pseudo-element of a list item:
ul li::marker {
color: red;
}
We can’t just select a pseudo-element using the document.querySelector()
method, but you can get the computed styles like this:
const element = document.querySelector('ul li');
const color = window.getComputedStyle(element, '::marker').color;
console.log(color); // rgb(255, 0, 0)
If you try to get styles for “an older pseudo-element” like the ::before
pseudo-element, you can also use :before
, but you can’t use :marker
, as this is a newer pseudo-element, for which the variant with just one colon does not exist.
In this code snippet, I’m not using the getPropertyValue()
, as it’s not strictly necessary. You can get most properties directly by their names. I’d recommend taking a look at the CSSStyleDeclaration
object documentation of log the object to the console, to inspect the available properties.
What you can also see in this example is that we don’t get the value red
, we have used in our CSS. Instead, we get rgb(255, 0, 0)
as the computed style. You can find some more information on this in the notes on the documentation page.
Conclusion
You might have some JavaScript code that needs to know the styles of an element. Let it be the size, color, position or other properties that would have an effect on your code. In these cases, the getComputedStyle()
is really helpful. WordPress Core is also using it in different place, like in the color-picker to warn about a too low color contrast. That’s a very good example on how to use the function.